Description
An Android UI library that allows easy implementation of (text) input suggestion popup windows.
Suggestive ๐ alternatives and similar packages
Based on the "Adapter" category.
Alternatively, view Suggestive ๐ alternatives based on common mentions on social networks and blogs.
-
FastAdapter
The bullet proof, fast and easy to use adapter library, which minimizes developing time to a fraction... -
SectionedRecyclerViewAdapter
DISCONTINUED. An Adapter that allows a RecyclerView to be split into Sections with headers and/or footers. Each Section can have its state controlled individually. -
Renderers
Renderers is an Android library created to avoid all the boilerplate needed to use a RecyclerView/ListView with adapters. -
SmartRecyclerAdapter
Small, smart and generic adapter for recycler view with easy and advanced data to ViewHolder binding. -
GridListViewAdapters
This library provides GridAdapters(ListGridAdapter & CursorGridAdapter) which enable you to bind your data in grid card fashion within android.widget.ListView, Also provides many other features related to GridListView. -
instant-adapter
DISCONTINUED. Just like instant coffee, saves 78% of your time on Android's Custom Adapters. -
EasyListViewAdapters
This library provides Easy Android ListView Adapters(EasyListAdapter & EasyCursorAdapter) which makes designing Multi-Row-Type ListView very simple & cleaner, It also provides many useful features for ListView. -
MultiLevelAdapter
Android library to allow collapsing and expanding items in RecyclerView's Adapter on multiple levels
InfluxDB - Power Real-Time Data Analytics at Scale
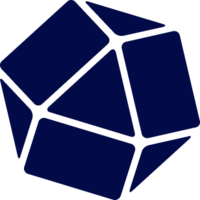
* Code Quality Rankings and insights are calculated and provided by Lumnify.
They vary from L1 to L5 with "L5" being the highest.
Do you think we are missing an alternative of Suggestive ๐ or a related project?
README
Suggestive ๐
An Android UI library that allows easy implementation of (text) input suggestion popup windows.
- Implement filtering using the onQuery callback
- Creates suggestion popups from RecyclerView adapters
- Creates suggestion popups from any View
- Works and animates well with soft keyboard visibility changes
- Automatically closes on back press if hosted in an AppCompatActivity
Gradle
Include the Bintray JCenter repository in the root build.gradle:
allprojects {
repositories {
maven {
url "https://jcenter.bintray.com"
}
}
}
Add the dependency to app build.gradle:
dependencies {
implementation 'dev.xtr.suggestive:suggestive:1.0.3'
}
Sample
Create a suggestion popup from a recycler adapter
To create a popup window of a RecyclerView use Suggestive.recycler()
.
All named arguments are optional and set to their default in this sample.
// Create a suggestion popup containing a recycler view
Suggestive.recycler(
// anchor to queryEditText
queryEditText,
// use the recycler view adapter
adapter,
// only invoke onQuery at a minimum input of 3 characters
minCharacters = 3,
// attaches the text change listener that invokes [onQuery]
attachTextChangeListener = true,
// invoke onQuery with a minimum delay of 200 ms
onQueryThrottle = 200,
// align the popup to the start of the anchor view
gravity = Gravity.START,
// use the background drawable for the popup window background
backgroundDrawable = ContextCompat.getDrawable(this, R.drawable.popup_rounded_bg)!!,
// constrains the popup windows width to the width of anchor
constrainWidthToAnchorBounds = true,
// hide the popup window when anchor loses focus
hideOnBlur = true,
// show the popup above or below anchor based on available space around anchor
preferredPosition = SuggestionWindow.PreferredPosition.BEST_FIT,
// dismisses the popup when the back button is pressed
dismissOnBackPress = true,
// the input query callback, called for every text change event on anchor
// (with exclusions as mandated by the minCharacters and onQueryThrottle options)
onQuery = { query ->
vm.search(query)
})
Create a suggestion popup from a view
To create a popup window of a view use Suggestive.view()
, this method takes the same arguments as recycler()
.
val view: View = //...
Suggestive.view(anchorView, view)
Manipulate the popup manually
Both factory methods on the Suggestive
object return instances of SuggestionWindow
.
Use methods on this object to manipulate the popup manually:
val popup = Suggestive.view(anchorView, view)
popup.show() // popup.isHidden = true
popup.hide() // popup.isHidden = false
popup.requestLayout() // relayout the popup content view
Demo
See the demo gif below or try it out on Google Play:
[Suggestive Demo GIF](demo.gif)