Android SegmentedControl + multi row support alternatives and similar packages
Based on the "UI Widget" category.
Alternatively, view Android SegmentedControl + multi row support alternatives based on common mentions on social networks and blogs.
-
EffectiveAndroidUI
Sample project created to show some of the best Android practices to work in the Android UI Layer. The UI layer of this project has been implemented using MVP or MVVM (without binding engine) to show how this patterns works. This project is used during the talk "EffectiveAndroidUI". -
ShowcaseView
DISCONTINUED. Highlight the best bits of your app to users quickly, simply, and cool...ly -
GreenDroid
GreenDroid is a development library for the Android platform. It makes UI developments easier and consistent through your applications. -
FancyToast-Android
Make your native android Toasts Fancy. A library that takes the standard Android toast to the next level with a variety of styling options. Style your toast from code. -
Smiley Rating
SmileyRating is a simple rating bar for android. It displays animated smileys as rating icon. -
ParallaxEverywhere
Parallax everywhere is a library with alternative android widgets with parallax effects. -
FancyAlertDialog-Android
Make your native android Dialog Fancy. A library that takes the standard Android Dialog to the next level with a variety of styling options. Style your dialog from code. -
MaterialBanner
A library that provides an implementation of the banner widget from the Material design. -
Custom-Calendar-View
The CustomCalendarView provides an easy and customizable calendar to create a Calendar. It dispaly the days of a month in a grid layout and allows to navigate between months -
Aesthetic GraphView
This is a custom graph library where you can customize the graph as you want. The key features are you can take the full control over drawing the path, change the gradient color (Start Color - End Color), Change the circle color, Change the circle radius, Change the path color, Change the line thickness, On/Off Gridlines, Change the grid line color, On/Off Graduations, Change the graduation text color, Draw graph with different starting point, Draw graph from the left border (X0 - coordinate), Draw graph with exact coordinates given, Draw graph from left border and stretch until the end of the screen and it is Supported on OS - JellyBean 4.1 and above -
FingerSignView
A simple library to let you sign (or draw lines) smoothly with your finger into a view and save it. -
Horizontal Calendar View
A simple library to display a horizontal calendar with custom start and end date, and mark events with a background
WorkOS - The modern identity platform for B2B SaaS
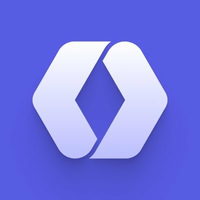
* Code Quality Rankings and insights are calculated and provided by Lumnify.
They vary from L1 to L5 with "L5" being the highest.
Do you think we are missing an alternative of Android SegmentedControl + multi row support or a related project?
Popular Comparisons
-
Android SegmentedControl + multi row supportvsCircleControlView
-
Android SegmentedControl + multi row supportvsandroid-ui
-
Android SegmentedControl + multi row supportvsProSwipeButton
-
Android SegmentedControl + multi row supportvsGIFView
-
Android SegmentedControl + multi row supportvsSmiley Rating
README
Android SegmentedControl + multi row support + multi selection
minSdk API 14+
Segmented control for Android, with a lot of customization properties
ScreenShots
Download
Gradle
Add to project level build.gradle
allprojects {
repositories {
...
maven { url 'https://jitpack.io' }
}
}
Add dependency to app module level build.gradle
dependencies {
implementation 'com.github.RobertApikyan:SegmentedControl:1.2.0'
}
Maven
<repositories>
<repository>
<id>jitpack.io</id>
<url>https://jitpack.io</url>
</repository>
</repositories>
Add dependency
<dependency>
<groupId>com.github.RobertApikyan</groupId>
<artifactId>SegmentedControl</artifactId>
<version>1.1.3</version>
</dependency>
Done.
Simple usage in XML
<segmented_control.widget.custom.android.com.segmentedcontrol.SegmentedControl
android:id="@+id/segmented_control"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:layout_margin="8dp"
app:columnCount="3"
app:distributeEvenly="true"
app:textVerticalPadding="6dp"
app:radius="12dp"
app:segments="@array/your_array_data" />
Attributes
<attr name="supportedSelectionsCount" format="boolean" /> setSupportedSelectionsCount(int)
<attr name="reselectionEnabled" format="boolean" /> setDistributeEvenly(boolean)
<attr name="distributeEvenly" format="boolean" /> setDistributeEvenly(boolean)
<attr name="columnCount" format="integer" /> setColumnCount(int)
<attr name="segments" format="reference" /> addSegments(Object[]), addSegments(List)
<attr name="selectedStrokeColor" format="color" /> setSelectedStrokeColor(int)
<attr name="unSelectedStrokeColor" format="color" /> setUnSelectedStrokeColor(int)
<attr name="strokeWidth" format="dimension" / setStrokeWidth(int)
<attr name="selectedBackgroundColor" format="color" /> setSelectedBackgroundColor(int)
<attr name="unSelectedBackgroundColor" format="color" /> setUnSelectedBackgroundColor(int)
<attr name="selectedTextColor" format="color"/> setSelectedTextColor(int)
<attr name="unSelectedTextColor" format="color"/> setUnSelectedTextColor(int)
<attr name="textSize" format="dimension"/> setTextSize(int)
<attr name="selectionAnimationDuration" format="integer"/>
<attr name="focusedBackgroundColor" format="color"/>
<attr name="textHorizontalPadding" format="dimension"/> setTextHorizontalPadding(int)
<attr name="textVerticalPadding" format="dimension"/> setTextVerticalPadding(int)
<attr name="segmentVerticalMargin" format="dimension"/> setSegmentVerticalMargin(int)
<attr name="segmentHorizontalMargin" format="dimension"/> setSegmentHorizontalMargin(int)
<attr name="radius" format="dimension"/> setRadius(int)
<attr name="topLeftRadius" format="dimension"/> setTopLeftRadius(int)
<attr name="topRightRadius" format="dimension"/> setTopRightRadius(int)
<attr name="bottomRightRadius" format="dimension"/> setBottomRightRadius(int)
<attr name="bottomLeftRadius" format="dimension"/> setBottomLeftRadius(int)
<attr name="radiusForEverySegment" format="boolean"/> setRadiusForEverySegment(boolean)
<attr name="fontAssetPath" format="string"/> setTypeFace(TypeFace)
Note: After every configuration change call segmentedControl.notifyConfigIsChanged() method
Example
segmentedControl.setStrokeWidth(width.intValue());
segmentedControl.setColumnCount(columnCount);
segmentedControl.notifyConfigIsChanged();
SegmentedControl uses SegmentAdapter and SegmentViewHolder for displaying segments. There are allready exist a default implementations for SegmentAdapter (SegmentAdapterImpl) and SegmentViewHolder (SegmentViewHolderImpl), but if you want to make your custom implementation... well here is the steps
Define
segment_item.xml
insidelayouts
folder<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="match_parent" android:layout_height="match_parent" android:orientation="vertical"> <TextView android:id="@+id/text_view" android:layout_width="match_parent" android:layout_height="match_parent" android:layout_gravity="center" android:layout_margin="2dp" android:background="@color/colorPrimary" android:gravity="center" android:textColor="@color/colorAccent" android:textSize="14sp" /> </LinearLayout>
Create a
SegmentViewHolder
instance (AppSegmentViewHolder
) (here I define the segment generic data type as a String)public class AppSegmentViewHolder extends SegmentViewHolder<String> { TextView textView; public AppSegmentViewHolder(@NonNull View sectionView) { super(sectionView); textView = (TextView) sectionView.findViewById(R.id.text_view); } @Override protected void onSegmentBind(String segmentData) { textView.setText(segmentData); } }
Create a
SegmentAdapter
instancepublic class AppSegmentAdapter extends SegmentAdapter<String, AppSegmentViewHolder> { @NonNull @Override protected AppSegmentViewHolder onCreateViewHolder(@NonNull LayoutInflater layoutInflater, ViewGroup viewGroup, int i) { return new AppSegmentViewHolder(layoutInflater.inflate(R.layout.item_segment, null)); } }
Pass the adapter to the segmentedControl
segmentedControl = (SegmentedControl) findViewById(R.id.segmented_control); segmentedControl.setAdapter(new AppSegmentAdapter());
Finally add segments data.
segmentedControl.addSegments(getResources().getStringArray(R.array.segments));
That's it :)
For custom implementation use SegmentedControlUtils
helper class in order to define segment background type and background radius.
License
Copyright 2017 Robert Apikyan
Licensed under the Apache License, Version 2.0 (the "License");
you may not use this file except in compliance with the License.
You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software
distributed under the License is distributed on an "AS IS" BASIS,
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
See the License for the specific language governing permissions and
limitations under the License.
*Note that all licence references and agreements mentioned in the Android SegmentedControl + multi row support README section above
are relevant to that project's source code only.