Anko alternatives and similar packages
Based on the "Kotlin" category.
Alternatively, view Anko alternatives based on common mentions on social networks and blogs.
-
Balloon
:balloon: Modernized and sophisticated tooltips, fully customizable with an arrow and animations for Android. -
kotlin-android-template
Android + Kotlin + Github Actions + ktlint + Detekt + Gradle Kotlin DSL + buildSrc = โค๏ธ -
NotyKT ๐๏ธ
๐ NotyKT is a complete ๐Kotlin-stack (Backend + Android) ๐ฑ application built to demonstrate the use of Modern development tools with best practices implementation๐ฆธ. -
Material Chip View
Material Chip view. Can be used as tags for categories, contacts or creating text clouds -
DrawableToolbox
๐ ๏ธ The missing drawable toolbox for Android. Create drawables programmatically and get rid of the boring and always repeated drawable.xml files. -
Navigation Toolbar for Android
:octocat: Navigation toolbar is a slide-modeled UI navigation controller made by @Ramotion -
Capturable
๐Jetpack Compose utility library for capturing Composable content and transforming it into Bitmap Image๐ผ๏ธ -
Pdf Viewer For Android
A Lightweight PDF Viewer Android library which only occupies around 80kb while most of the Pdf viewer occupies up to 16MB space. -
Carousel Recyclerview
Carousel Recyclerview let's you create carousel layout with the power of recyclerview by creating custom layout manager. -
Pluto Debug Framework
Android Pluto is a on-device debugging framework for Android applications, which helps intercept Network calls, capture Crashes & ANRs, manipulate application data on-the-go, and much more. -
SSComposeCookBook
A Collection of major Jetpack compose UI components which are commonly used.๐๐๐ -
CrunchyCalendar โ awesome calendar widget for android apps
A beautiful material calendar with endless scroll, range selection and a lot more! -
SSCustomBottomNavigation
Animated TabBar with native control and Jetpack Navigation support..โจ๐๐ -
Permission Flow for Android
Know about real-time state of a Android app Permissions with Kotlin Flow APIs. -
Only
:bouquet: An easy way to persist and run code block only as many times as necessary on Android. -
Nextflix-Composable
Includes jetpack compose, navigation, paging, hilt, retrofit, coil, coroutines, flow.. -
EasyPermissions-ktx
๐ Kotlin version of the popular google/easypermissions wrapper library to simplify basic system permissions logic on Android M or higher. -
Compose Compiler Reports to HTML Generator
A utility (Gradle Plugin + CLI) to convert Jetpack Compose compiler metrics and reports to beautified HTML page. -
Events Calendar
Events Calendar is a user-friendly library that helps you achieve a cool Calendar UI with events mapping. You can customise every pixel of the calendar as per your wish and still achieve in implementing all the functionalities of the native android calendar in addition with adding dots to the calendar which represents the presence of an event on the respective dates. It can be done easily, you are just a few steps away from implementing your own badass looking Calendar for your very own project! -
MidJourney Images Compose Multiplatform Mobile Application
This application is developed to display the images created by MidJourney. The application is developed with Compose Multiplatform and works on many platforms including Android and iOS platforms. -
SSCustomEditTextOutLineBorder
Same as the Outlined text fields presented on the Material Design page but with some dynamic changes. ๐ ๐ -
TimelineView
A customizable and easy-to-use Timeline View library for Android. Works as a RecyclerView decorator (ItemDecoration) -
Vanilla Place Picker
Simple(vanilla) yet 'Do it all' place picker for your place picking needs in Android
WorkOS - The modern identity platform for B2B SaaS
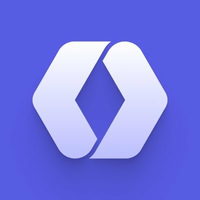
Do you think we are missing an alternative of Anko or a related project?
README
:warning: Anko is deprecated. Please see this page for more information.
Anko is a Kotlin library which makes Android application development faster and easier. It makes your code clean and easy to read, and lets you forget about rough edges of the Android SDK for Java.
Anko consists of several parts:
- Anko Commons: a lightweight library full of helpers for intents, dialogs, logging and so on;
- Anko Layouts: a fast and type-safe way to write dynamic Android layouts;
- Anko SQLite: a query DSL and parser collection for Android SQLite;
- Anko Coroutines: utilities based on the kotlinx.coroutines library.
Anko Commons
Anko Commons is a "toolbox" for Kotlin Android developer. The library contains a lot of helpers for Android SDK, including, but not limited to:
Anko Layouts (wiki)
Anko Layouts is a DSL for writing dynamic Android layouts. Here is a simple UI written with Anko DSL:
verticalLayout {
val name = editText()
button("Say Hello") {
onClick { toast("Hello, ${name.text}!") }
}
}
The code above creates a button inside a LinearLayout
and attaches an OnClickListener
to that button. Moreover, onClick
accepts a suspend
lambda, so you can write your asynchronous code right inside the listener!
Note that this is the complete layout code. No XML is required!
Anko has a DSL for ConstraintLayout since v0.10.4
There is also a plugin for Android Studio that supports previewing Anko DSL layouts.
Anko SQLite (wiki)
Have you ever been tired of parsing SQLite query results using Android cursors? Anko SQLite provides lots of helpers to simplify working with SQLite databases.
For example, here is how you can fetch the list of users with a particular name:
fun getUsers(db: ManagedSQLiteOpenHelper): List<User> = db.use {
db.select("Users")
.whereSimple("family_name = ?", "John")
.doExec()
.parseList(UserParser)
}
Anko Coroutines (wiki)
Anko Coroutines is based on the kotlinx.coroutines
library and provides:
bg()
function that executes your code in a common pool.asReference()
function which creates a weak reference wrapper. By default, a coroutine holds references to captured objects until it is finished or canceled. If your asynchronous framework does not support cancellation, the values you use inside the asynchronous block can be leaked.asReference()
protects you from this.
Using Anko
Gradle-based project
Anko has a meta-dependency which plugs in all available features (including Commons, Layouts, SQLite) into your project at once:
dependencies {
implementation "org.jetbrains.anko:anko:$anko_version"
}
Make sure that you have the $anko_version
settled in your gradle file at the project level:
ext.anko_version='0.10.8'
If you only need some of the features, you can reference any of Anko's parts:
dependencies {
// Anko Commons
implementation "org.jetbrains.anko:anko-commons:$anko_version"
// Anko Layouts
implementation "org.jetbrains.anko:anko-sdk25:$anko_version" // sdk15, sdk19, sdk21, sdk23 are also available
implementation "org.jetbrains.anko:anko-appcompat-v7:$anko_version"
// Coroutine listeners for Anko Layouts
implementation "org.jetbrains.anko:anko-sdk25-coroutines:$anko_version"
implementation "org.jetbrains.anko:anko-appcompat-v7-coroutines:$anko_version"
// Anko SQLite
implementation "org.jetbrains.anko:anko-sqlite:$anko_version"
}
There are also a number of artifacts for the Android support libraries:
dependencies {
// Appcompat-v7 (only Anko Commons)
implementation "org.jetbrains.anko:anko-appcompat-v7-commons:$anko_version"
// Appcompat-v7 (Anko Layouts)
implementation "org.jetbrains.anko:anko-appcompat-v7:$anko_version"
implementation "org.jetbrains.anko:anko-coroutines:$anko_version"
// CardView-v7
implementation "org.jetbrains.anko:anko-cardview-v7:$anko_version"
// Design
implementation "org.jetbrains.anko:anko-design:$anko_version"
implementation "org.jetbrains.anko:anko-design-coroutines:$anko_version"
// GridLayout-v7
implementation "org.jetbrains.anko:anko-gridlayout-v7:$anko_version"
// Percent
implementation "org.jetbrains.anko:anko-percent:$anko_version"
// RecyclerView-v7
implementation "org.jetbrains.anko:anko-recyclerview-v7:$anko_version"
implementation "org.jetbrains.anko:anko-recyclerview-v7-coroutines:$anko_version"
// Support-v4 (only Anko Commons)
implementation "org.jetbrains.anko:anko-support-v4-commons:$anko_version"
// Support-v4 (Anko Layouts)
implementation "org.jetbrains.anko:anko-support-v4:$anko_version"
// ConstraintLayout
implementation "org.jetbrains.anko:anko-constraint-layout:$anko_version"
}
There is an example project showing how to include Anko library into your Android Gradle project.
IntelliJ IDEA project
If your project is not based on Gradle, just attach the required JARs from the jcenter repository as the library dependencies and that's it.
Contributing
The best way to submit a patch is to send us a pull request. Before submitting the pull request, make sure all existing tests are passing, and add the new test if it is required.
If you want to add new functionality, please file a new proposal issue first to make sure that it is not in progress already. If you have any questions, feel free to create a question issue.
Instructions for building Anko are available in the Wiki.
*Note that all licence references and agreements mentioned in the Anko README section above
are relevant to that project's source code only.