Description
๐ An easy way to persist and run code block only as many times as necessary on Android.
Only alternatives and similar packages
Based on the "Kotlin" category.
Alternatively, view Only alternatives based on common mentions on social networks and blogs.
-
Balloon
:balloon: Modernized and sophisticated tooltips, fully customizable with an arrow and animations for Android. -
kotlin-android-template
Android + Kotlin + Github Actions + ktlint + Detekt + Gradle Kotlin DSL + buildSrc = โค๏ธ -
NotyKT ๐๏ธ
๐ NotyKT is a complete ๐Kotlin-stack (Backend + Android) ๐ฑ application built to demonstrate the use of Modern development tools with best practices implementation๐ฆธ. -
Material Chip View
Material Chip view. Can be used as tags for categories, contacts or creating text clouds -
DrawableToolbox
๐ ๏ธ The missing drawable toolbox for Android. Create drawables programmatically and get rid of the boring and always repeated drawable.xml files. -
Navigation Toolbar for Android
:octocat: Navigation toolbar is a slide-modeled UI navigation controller made by @Ramotion -
Capturable
๐Jetpack Compose utility library for capturing Composable content and transforming it into Bitmap Image๐ผ๏ธ -
Pdf Viewer For Android
A Lightweight PDF Viewer Android library which only occupies around 80kb while most of the Pdf viewer occupies up to 16MB space. -
Carousel Recyclerview
Carousel Recyclerview let's you create carousel layout with the power of recyclerview by creating custom layout manager. -
Pluto Debug Framework
Android Pluto is a on-device debugging framework for Android applications, which helps intercept Network calls, capture Crashes & ANRs, manipulate application data on-the-go, and much more. -
CrunchyCalendar โ awesome calendar widget for android apps
A beautiful material calendar with endless scroll, range selection and a lot more! -
SSComposeCookBook
A Collection of major Jetpack compose UI components which are commonly used.๐๐๐ -
Permission Flow for Android
Know about real-time state of a Android app Permissions with Kotlin Flow APIs. -
SSCustomBottomNavigation
Animated TabBar with native control and Jetpack Navigation support..โจ๐๐ -
Nextflix-Composable
Includes jetpack compose, navigation, paging, hilt, retrofit, coil, coroutines, flow.. -
EasyPermissions-ktx
๐ Kotlin version of the popular google/easypermissions wrapper library to simplify basic system permissions logic on Android M or higher. -
Compose Compiler Reports to HTML Generator
A utility (Gradle Plugin + CLI) to convert Jetpack Compose compiler metrics and reports to beautified HTML page. -
Events Calendar
Events Calendar is a user-friendly library that helps you achieve a cool Calendar UI with events mapping. You can customise every pixel of the calendar as per your wish and still achieve in implementing all the functionalities of the native android calendar in addition with adding dots to the calendar which represents the presence of an event on the respective dates. It can be done easily, you are just a few steps away from implementing your own badass looking Calendar for your very own project! -
MidJourney Images Compose Multiplatform Mobile Application
This application is developed to display the images created by MidJourney. The application is developed with Compose Multiplatform and works on many platforms including Android and iOS platforms. -
SSCustomEditTextOutLineBorder
Same as the Outlined text fields presented on the Material Design page but with some dynamic changes. ๐ ๐ -
TimelineView
A customizable and easy-to-use Timeline View library for Android. Works as a RecyclerView decorator (ItemDecoration) -
Vanilla Place Picker
Simple(vanilla) yet 'Do it all' place picker for your place picking needs in Android
InfluxDB - Power Real-Time Data Analytics at Scale
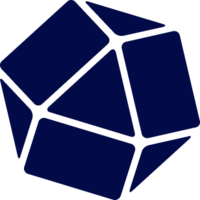
Do you think we are missing an alternative of Only or a related project?
Popular Comparisons
README
Only
:bouquet: An easy way to persist and run code block only as many times as necessary on Android.
Download
Gradle
Add a dependency code to your module's build.gradle
file.
dependencies {
implementation "com.github.skydoves:only:1.0.7"
}
Usage
Initialize
First, initialize the Only
using init()
method like below.
This code can be initialized on Application
class only once.
Only.init(context)
onDo
Below codes will run the showIntroPopup()
only three times using onDo
method.
Only.onDo("introPopup", times = 3) {
showIntroPopup()
}
// kotlin dsl
only("introPopup", times = 3) {
onDo { showIntroPopup() }
}
Here is the Java codes.
Only.onDo("introPopup", 1, () -> showIntroPopup());
onDone
Below codes will run the doSomethingAfterDone()
and toast("done")
after run the onDo
block codes three times.
Only.onDo("introPopup", times = 3,
onDo = {
showIntroPopup()
toast("onDo only three times")
},
onDone = {
doSomethingAfterDone()
toast("done")
})
// kotlin dsl
only("introPopup", times = 3) {
onDo {
showIntroPopup()
toast("onDo only three times")
}
onDone {
doSomeThingAfterDone()
toast("done")
}
}
Here is the Java codes.
Only.onDo("introPopup", 1,
() -> doSomethingOnlyOnce(), // onDo
() -> doSOmethingAfterDone() // onDone
);
onLastDo, onBeforeDone
We can do pre and post-processing using onLastDo
, onBeforeDone
options.
only("Intro", times = 3) {
onDo {
showIntroPopup()
toast("onDo only three times")
}
onLastDo { // executes only once after finished onDo block 3 times.
toast("finished onDo")
}
onBeforeDone { // executes only once before run onDone block.
toast("starts onDo")
}
onDone {
doSomethingAfterDone()
toast("done")
}
}
We can apply it for repeating x times.
Below codes shows review-popup 3 times and checks the user reviewed or not in onLastDo
block.
If not, clear times using the Only.clearOnly
method, and repeat it the first time again.
only("Intro", times = 3) {
onDo { showReviewRequestPopup() }
onLastDo { // executes only once after finished onDo block 3 times.
if (!isRequested) {
Only.clearOnly([email protected])
}
}
}
Version Control
We can renew the persistence times for controlling the version using version
option.
If the version is different from the old version, run times will be initialized 0.
Only.onDo("introPopup", times = 3,
onDo = { showIntroPopup() },
onDone = { doSomethingAfterDone() },
version = BuildConfig.VERSION_NAME // we can set manually. e.g. "1.1.1.1"
)
// kotlin dsl
only("introPopup", times = 3) {
onDo { showIntroPopup() }
onDone { doSomethingAfterDone() }
version("1.1.1.1")
}
Create Using Builder
We can run Only using Only.Builder
class like below.
Only.Builder("introPopup", times = 3)
.onDo { showIntroPopup() }
.onDone { doSomethingAfterDone() }
.version(BuildConfig.VERSION_NAME)
.run()
OnlyOnce, OnlyTwice, OnlyThrice
Here is some useful kotlin-dsl functions.
onlyOnce("onlyOnce") { // run the onDo block codes only once.
onDo { doSomethingOnlyOnce() }
onDone { doSomethingAfterDone() }
}
onlyTwice("onlyTwice") { // run the onDo block codes only twice.
onDo { doSomethingOnlyTwice() }
onDone { doSomethingAfterDone() }
}
onlyThrice("onlyThrice") { // run the onDo block codes only three times.
onDo { doSomethingOnlyThrice() }
onDone { doSomethingAfterDone() }
version("1.1.1.1")
}
Clear Data
We can optionally delete the stored Only
target data or delete the whole Only
data.
Only.clearOnly("introPopup") // clears a saved target Only data.
Only.clearAllOnly() // clears all of the target Only data on the application.
View Extension
Below codes will show the button
view only once.
button.onlyVisibility(name = "myButton", times = 1, visible = true)
Toast Extension
Below codes will show toast only x times.
onlyToast("toast", 3, "This toast will be shown only three times.")
onlyOnceToast("toast1", "This toast will be shown only once.")
onlyTwiceToast("toast2", "This toast will be shown only twice.")
onlyThriceToast("toast3", "This toast will be shown only thrice.")
Marking
We can mark data to the Only target.
only("introPopup", times = 3) {
onDo { showIntroPopup() }
onDone { doSomethingAfterDone() }
mark("abc") // marks only once when run by kotlin dsl or builder class.
}
Only.mark("introPopup", 3) // changes marking using mark method.
val marking = Only.getMarking("introPopup") // gets the marked data.
Debug Mode
Sometimes on debug, we don't need to persist data and replay onDone block.
onlyOnDoDebugMode
helps that ignore persistence data and onDone block when initialization. It runs only onDo block.
val only = Only.init(application)
if (BuildConfig.DEBUG) {
only.onlyOnDoDebugMode(true)
}
Usage in Java
Here are some usages for Java developers.
int times = Only.getOnlyTimes("IntroPopup") ;
if (times < 3) {
Only.setOnlyTimes("IntroPopup", times + 1);
showIntroPopup();
}
Java Supports
we can run Only
in our java project.
Only.onDo("introPopup", 1,
new Runnable() {
@Override
public void run() {
doSomethingOnlyOnce();
}
}, new Runnable() {
@Override
public void run() {
doSOmethingAfterDone();
}
});
Or we can run using Only.Builder
like below.
new Only.Builder("introPopup", 1)
.onDo(new Runnable() {
@Override
public void run() {
doSomethingOnlyOnce();
}
})
.onDone(new Runnable() {
@Override
public void run() {
doSOmethingAfterDone();
}
}).run(); // run the Only
Java8 lambda expression
We can make it more simple using Java8 lambda expression.
Add below codes on your build.gradle
file.
android {
compileOptions {
sourceCompatibility JavaVersion.VERSION_1_8
targetCompatibility JavaVersion.VERSION_1_8
}
}
Then you can run the Only like below.
Only.onDo("introPopup", 1,
() -> doSomethingOnlyOnce(), // onDo
() -> doSOmethingAfterDone() // onDone
);
Custom util class
We can create custom util class like what Kotlin's onlyOnce
.
public class OnlyUtils {
public static void onlyOnce(
String name, Runnable runnableOnDo, Runnable runnableOnDone) {
new Only.Builder(name, 1)
.onDo(runnableOnDo)
.onDone(runnableOnDone)
.run(); // run the Only
}
}
Find this library useful? :heart:
Support it by joining stargazers for this repository. :star: And follow me for my next creations! ๐คฉ
License
Copyright 2019 skydoves (Jaewoong Eum)
Licensed under the Apache License, Version 2.0 (the "License");
you may not use this file except in compliance with the License.
You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software
distributed under the License is distributed on an "AS IS" BASIS,
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
See the License for the specific language governing permissions and
limitations under the License.
*Note that all licence references and agreements mentioned in the Only README section above
are relevant to that project's source code only.