Time alternatives and similar packages
Based on the "Kotlin" category.
Alternatively, view Time alternatives based on common mentions on social networks and blogs.
-
Balloon
:balloon: Modernized and sophisticated tooltips, fully customizable with an arrow and animations for Android. -
kotlin-android-template
Android + Kotlin + Github Actions + ktlint + Detekt + Gradle Kotlin DSL + buildSrc = โค๏ธ -
NotyKT ๐๏ธ
๐ NotyKT is a complete ๐Kotlin-stack (Backend + Android) ๐ฑ application built to demonstrate the use of Modern development tools with best practices implementation๐ฆธ. -
Material Chip View
Material Chip view. Can be used as tags for categories, contacts or creating text clouds -
DrawableToolbox
๐ ๏ธ The missing drawable toolbox for Android. Create drawables programmatically and get rid of the boring and always repeated drawable.xml files. -
Navigation Toolbar for Android
:octocat: Navigation toolbar is a slide-modeled UI navigation controller made by @Ramotion -
Capturable
๐Jetpack Compose utility library for capturing Composable content and transforming it into Bitmap Image๐ผ๏ธ -
Pdf Viewer For Android
A Lightweight PDF Viewer Android library which only occupies around 80kb while most of the Pdf viewer occupies up to 16MB space. -
Carousel Recyclerview
Carousel Recyclerview let's you create carousel layout with the power of recyclerview by creating custom layout manager. -
Pluto Debug Framework
Android Pluto is a on-device debugging framework for Android applications, which helps intercept Network calls, capture Crashes & ANRs, manipulate application data on-the-go, and much more. -
CrunchyCalendar โ awesome calendar widget for android apps
A beautiful material calendar with endless scroll, range selection and a lot more! -
SSComposeCookBook
A Collection of major Jetpack compose UI components which are commonly used.๐๐๐ -
SSCustomBottomNavigation
Animated TabBar with native control and Jetpack Navigation support..โจ๐๐ -
Permission Flow for Android
Know about real-time state of a Android app Permissions with Kotlin Flow APIs. -
Only
:bouquet: An easy way to persist and run code block only as many times as necessary on Android. -
Nextflix-Composable
Includes jetpack compose, navigation, paging, hilt, retrofit, coil, coroutines, flow.. -
EasyPermissions-ktx
๐ Kotlin version of the popular google/easypermissions wrapper library to simplify basic system permissions logic on Android M or higher. -
Compose Compiler Reports to HTML Generator
A utility (Gradle Plugin + CLI) to convert Jetpack Compose compiler metrics and reports to beautified HTML page. -
Events Calendar
Events Calendar is a user-friendly library that helps you achieve a cool Calendar UI with events mapping. You can customise every pixel of the calendar as per your wish and still achieve in implementing all the functionalities of the native android calendar in addition with adding dots to the calendar which represents the presence of an event on the respective dates. It can be done easily, you are just a few steps away from implementing your own badass looking Calendar for your very own project! -
MidJourney Images Compose Multiplatform Mobile Application
This application is developed to display the images created by MidJourney. The application is developed with Compose Multiplatform and works on many platforms including Android and iOS platforms. -
SSCustomEditTextOutLineBorder
Same as the Outlined text fields presented on the Material Design page but with some dynamic changes. ๐ ๐ -
TimelineView
A customizable and easy-to-use Timeline View library for Android. Works as a RecyclerView decorator (ItemDecoration) -
Vanilla Place Picker
Simple(vanilla) yet 'Do it all' place picker for your place picking needs in Android
WorkOS - The modern identity platform for B2B SaaS
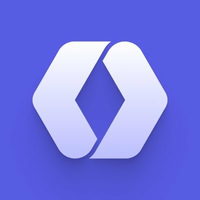
Do you think we are missing an alternative of Time or a related project?
README
Time
This library is made for you if you have ever written something like this:
val duration = 10 * 1000
to represent a duration of 10 seconds(in milliseconds) because most methods in Kotlin/Java take duration parameters in milliseconds.
Usage
Showcase
val tenSeconds = 10.seconds
val fiveMinutes = 5.minutes
val twoHours = 2.hours
val threeDays = 3.days
val tenMinutesFromNow = Calendar.getInstance() + 10.minutes
val tenSecondsInMilliseconds = 10.seconds.inMilliseconds
Basics
The main advantage of the library is that all time units are strongly-typed. So, for example:
val tenMinutes = 10.minutes
In the example above, tenMinutes
will be of type Interval<Minute>
. There are seven time units available, from nanoseconds to days:
val tenNanoseconds = 10.nanoseconds
// type is Interval<Nanosecond>
val tenMicroseconds = 10.microseconds
// type is Interval<Microsecond>
val tenMilliseconds = 10.milliseconds
// type is Interval<Millisecond>
val tenSeconds = 10.seconds
// type is Interval<Second>
val tenMinutes = 10.minutes
// type is Interval<Minute>
val tenHours = 10.hours
// type is Interval<Hour>
val tenDays = 10.days
// type is Interval<Day>
Operations
You can perform all basic arithmetic operations on time intervals, even of different units:
val duration = 10.minutes + 15.seconds - 3.minutes + 2.hours // Interval<Minute>
val doubled = duration * 2
val seconds = 10.seconds + 3.minutes // Interval<Second>
You can also use these operations with the Calendar
class:
val twoHoursLater = Calendar.getInstance() + 2.hours
Conversions
Time intervals are easily convertible:
val twoMinutesInSeconds = 2.minutes.inSeconds // Interval<Second>
val fourDaysInHours = 4.days.inHours // Interval<Hour>
You can also use the converted()
method, although you would rarely need to:
val tenMinutesInSeconds: Interval<Second> = 10.minutes.converted()
Comparison
You can compare different time units as well:
50.seconds < 2.hours // true
120.minutes == 2.hours // true
100.milliseconds > 2.seconds // false
48.hours in 2.days // true
Creating your own time units
If, for some reason, you need to create your own time unit, that's super easy to do:
class Week : TimeUnit {
// number of seconds in one week
override val timeIntervalRatio = 604800.0
}
Now you can use it like any other time unit:
val fiveWeeks = Interval<Week>(5)
For the sake of convenience, don't forget to write those handy extensions:
class Week : TimeUnit {
override val timeIntervalRatio = 604800.0
}
val Number.weeks: Interval<Week>
get() = Interval(this)
val Interval<TimeUnit>.inWeeks: Interval<Week>
get() = converted()
Now you can write:
val fiveWeeks = 5.weeks // Interval<Week>
You can also easily convert to weeks:
val valueInWeeks = 14.days.inWeeks // Interval<Week>
Extras
The library includes some handy extensions for some classes:
val now = Calendar.getInstance()
val sixHoursLater = now + 6.hours
val fourDaysAgo = now - 4.days
val timer = Timer()
timer.schedule(10.seconds) {
println("This block will be called in 10 seconds")
}
The library also includes extensions for Android's Handler class, this is only available if you compile the "time-android" module.
val handler = Handler()
handler.postDelayed({
Log.i("TAG", "This will be printed to the Logcat in 2 minutes")
}, 2.minutes)
More extensions will be added to the library in the future.
Conversion safety everywhere
For time-related methods in other third-party libraries in your project, if such methods are frequently used, it's best to write extention functions that let you use the time units in this libary in those methods. This is mostly just one line of code.
If such methods aren't frequently used, you can still benefit from the conversion safety that comes with this library.
An example method in a third-party library that does something after a delay period in milliseconds:
class Person {
fun doSomething(delayMillis: Long) {
// method body
}
}
To call the method above with a value of 5 minutes, one would usually write:
val person = Person()
person.doSomething(5 * 60 * 1000)
The above line can be written in a safer and clearer way using this library:
val person = Person()
person.doSomething(5.minutes.inMilliseconds.longValue)
If the method is frequently used, you can write an extension function:
fun Person.doSomething(delay: Interval<TimeUnit>) {
doSomething(delay.inMilliseconds.longValue)
}
Now you can write:
val person = Person()
person.doSomething(5.minutes)
Installation
Add the JitPack repository to your build.gradle
:
allprojects {
repositories {
maven { url "https://jitpack.io" }
}
}
Add the dependency to your build.gradle
:
- For non-Android projects:
dependencies {
compile 'com.github.kizitonwose.time:time:<version>'
}
- For Android projects:
dependencies {
compile 'com.github.kizitonwose.time:time-android:<version>'
}
Contributing
The goal is for the library to be used wherever possible. If there are extension functions or features you think the library should have, feel free to add them and send a pull request or open an issue. Core Kotlin extensions belong in the "time" module while Android extensions belong in "time-android" module.
Inspiration
Time was inspired by a Swift library of the same name - Time.
The API of Time(Kotlin) has been designed to be as close as possible to Time(Swift) for consistency and because the two languages have many similarities. Check out Time(Swift) if you want a library with the same functionality in Swift.
License
Time is distributed under the MIT license. See LICENSE for details.
*Note that all licence references and agreements mentioned in the Time README section above
are relevant to that project's source code only.