CodeView alternatives and similar packages
Based on the "Kotlin" category.
Alternatively, view CodeView alternatives based on common mentions on social networks and blogs.
-
Balloon
:balloon: Modernized and sophisticated tooltips, fully customizable with an arrow and animations for Android. -
kotlin-android-template
Android + Kotlin + Github Actions + ktlint + Detekt + Gradle Kotlin DSL + buildSrc = ❤️ -
NotyKT 🖊️
📒 NotyKT is a complete 💎Kotlin-stack (Backend + Android) 📱 application built to demonstrate the use of Modern development tools with best practices implementation🦸. -
Material Chip View
Material Chip view. Can be used as tags for categories, contacts or creating text clouds -
DrawableToolbox
🛠️ The missing drawable toolbox for Android. Create drawables programmatically and get rid of the boring and always repeated drawable.xml files. -
Navigation Toolbar for Android
:octocat: Navigation toolbar is a slide-modeled UI navigation controller made by @Ramotion -
Capturable
🚀Jetpack Compose utility library for capturing Composable content and transforming it into Bitmap Image🖼️ -
Pdf Viewer For Android
A Lightweight PDF Viewer Android library which only occupies around 80kb while most of the Pdf viewer occupies up to 16MB space. -
Carousel Recyclerview
Carousel Recyclerview let's you create carousel layout with the power of recyclerview by creating custom layout manager. -
Pluto Debug Framework
Android Pluto is a on-device debugging framework for Android applications, which helps intercept Network calls, capture Crashes & ANRs, manipulate application data on-the-go, and much more. -
CrunchyCalendar — awesome calendar widget for android apps
A beautiful material calendar with endless scroll, range selection and a lot more! -
Permission Flow for Android
Know about real-time state of a Android app Permissions with Kotlin Flow APIs. -
Only
:bouquet: An easy way to persist and run code block only as many times as necessary on Android. -
Nextflix-Composable
Includes jetpack compose, navigation, paging, hilt, retrofit, coil, coroutines, flow.. -
EasyPermissions-ktx
🔓 Kotlin version of the popular google/easypermissions wrapper library to simplify basic system permissions logic on Android M or higher. -
Compose Compiler Reports to HTML Generator
A utility (Gradle Plugin + CLI) to convert Jetpack Compose compiler metrics and reports to beautified HTML page. -
Events Calendar
Events Calendar is a user-friendly library that helps you achieve a cool Calendar UI with events mapping. You can customise every pixel of the calendar as per your wish and still achieve in implementing all the functionalities of the native android calendar in addition with adding dots to the calendar which represents the presence of an event on the respective dates. It can be done easily, you are just a few steps away from implementing your own badass looking Calendar for your very own project! -
MidJourney Images Compose Multiplatform Mobile Application
This application is developed to display the images created by MidJourney. The application is developed with Compose Multiplatform and works on many platforms including Android and iOS platforms. -
SSCustomEditTextOutLineBorder
Same as the Outlined text fields presented on the Material Design page but with some dynamic changes. 📝 🎉 -
TimelineView
A customizable and easy-to-use Timeline View library for Android. Works as a RecyclerView decorator (ItemDecoration) -
Vanilla Place Picker
Simple(vanilla) yet 'Do it all' place picker for your place picking needs in Android
InfluxDB - Power Real-Time Data Analytics at Scale
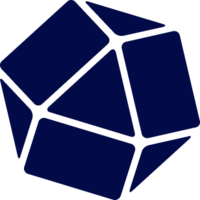
Do you think we are missing an alternative of CodeView or a related project?
README
CodeView (Android)
CodeView helps to show code content with syntax highlighting in native way.
Description
CodeView contains 3 core parts to implement necessary logic:
CodeView & related abstract adapter to provide options & customization (see below).
For highlighting it uses CodeHighlighter, it highlights your code & returns formatted content. It's based on Google Prettify and their Java implementation & fork.
CodeClassifier is trying to define what language is presented in the code snippet. It's built using Naive Bayes classifier upon found open-source implementation, which I rewrote in Kotlin. There is no need to work with this class directly & you must just follow instructions below. (Experimental module, may not work properly!)
Download
Add it in your root build.gradle
at the end of repositories:
allprojects {
repositories {
...
maven { url "https://jitpack.io" }
}
}
Add the dependency:
compile 'com.github.kbiakov:CodeView-Android:1.3.2'
Usage
If you want to use code classifier to auto language recognizing just add to your Application.java
:
// train classifier on app start
CodeProcessor.init(this);
Having done ones on app start you can classify language for different snippets even faster, because the algorithm needs time for training on sets for the presented listings of the languages which the library has.
Add view to your layout & bind as usual:
<io.github.kbiakov.codeview.CodeView
android:id="@+id/code_view"
android:layout_width="wrap_content"
android:layout_height="wrap_content" />
CodeView codeView = (CodeView) findViewById(R.id.code_view);
So now you can set code using implicit form:
// auto language recognition
codeView.setCode(getString(R.string.listing_js));
Or explicit (see available extensions below):
// will work faster!
codeView.setCode(getString(R.string.listing_py), "py");
Customization
When you call setCode(...)
the view will be prepared with the default params if the view was not initialized before. So if you want some customization, it can be done using the options and/or adapter.
Initialization
You can initialize the view with options:
codeView.setOptions(Options.Default.get(this)
.withLanguage("python")
.withCode(R.string.listing_py)
.withTheme(ColorTheme.MONOKAI));
Or using adapter (see Adapter or example for more details):
final CustomAdapter myAdapter = new CustomAdapter(this, getString(R.string.listing_md));
codeView.setAdapter(myAdapter);
Note: Each CodeView has a adapter and each adapter has options. When calling setOptions(...)
or setAdapter(...)
the current adapter is "flushed" with the current options. If you want to save the state and just update options saving adapter or set adapter saving options you must call updateOptions(...)
or updateAdapter(...)
accordingly.
Options
Options helps to easily set necessary params, such as code & language, color theme, font, format, shortcut params (max lines, note) and code line click listener. Some params are unnecessary.
When the view is initialized (options or adapter are set) you can manipulate the options in various ways:
codeView.getOptions()
.withCode(R.string.listing_java)
.withLanguage("java")
.withTheme(ColorTheme.MONOKAI);
Color theme
There are some default themes (see full list below):
codeView.getOptions().setTheme(ColorTheme.SOLARIZED_LIGHT);
But you can build your own from a existing one:
ColorThemeData myTheme = ColorTheme.SOLARIZED_LIGHT.theme()
.withBgContent(android.R.color.black)
.withNoteColor(android.R.color.white);
codeView.getOptions().setTheme(myTheme);
Or create your own from scratch (don't forget to open PR with this stuff!):
ColorThemeData customTheme = new ColorThemeData(new SyntaxColors(...), ...);
codeView.getOptions().setTheme(customTheme);
Font
Set font for your code content:
codeView.getOptions().withFont(Font.Consolas);
Font.Consolas
is a font preset (see the list of available below).
To use your own font you can use similar method by providing Typeface
or font path. Fonts are internally cached.
Format
Manage the space that code line take. There are 3 types: Compact
, ExtraCompact
and Medium
.
Setup is similar:
// Kotlin
codeView.getOptions().withFont(Font.Compact)
// Java
codeView.getOptions().withFont(Format.Default.getCompact());
Also you can create custom Format
by providing params such as scaleFactor
, lineHeight
, borderHeight
(above first line and below last) and fontSize
.
Adapter
Sometimes you may want to take code lines under your control, and that's why you need a Adapter.
You can create your own implementation as follows:
- Create your model to store data, for example some
MyModel
class. - Extend
AbstractCodeAdapter<MyModel>
typed by your model class. Implement necessary methods in obtained
MyCodeAdapter
:// Kotlin class MyCodeAdapter : AbstractCodeAdapter<MyModel> { constructor(context: Context, content: String) : super(context, content) override fun createFooter(context: Context, entity: MyModel, isFirst: Boolean) = /* init & return your view here */ }
// Java public class MyCodeAdapter extends AbstractCodeAdapter<MyModel> { public CustomAdapter(@NotNull Context context, @NotNull String content) { // @see params in AbstractCodeAdapter super(context, content, true, 10, context.getString(R.string.show_all), null); } @NotNull @Override public View createFooter(@NotNull Context context, CustomModel entity, boolean isFirst) { return /* your initialized view here */; } }
Set custom adapter to your code view:
final MyCodeAdapter adapter = new MyCodeAdapter(this, getString(R.string.listing_py)); codeView.setAdapter(diffsAdapter);
Init footer entities to provide mapper from your model to view:
// it will add an addition diff to code line adapter.addFooterEntity(16, new MyModel(getString(R.string.py_addition_16), true)); // and this a deletion diff adapter.addFooterEntity(11, new MyModel(getString(R.string.py_deletion_11), false));
You can also add a multiple diff entities:
AbstractCodeAdapter<MyModel>.addFooterEntities(HashMap<Int, List<MyModel>> myEntities)
Here you must provide a map from code line numbers (started from 0) to list of line entities. It will be mapped by adapter to specified footer views.
See Github diff as example of my "best practice" implementation.
How it looks in app
See example.
List of available languages & their extensions
C/C++/Objective-C ("c"
, "cc"
, "cpp"
, "cxx"
, "cyc"
, "m"
), C# ("cs"
), Java ("java"
), Bash ("bash"
, "bsh"
, "csh"
, "sh"
), Python ("cv"
, "py"
, "python"
), Perl ("perl"
, "pl"
, "pm"
), Ruby ("rb"
, "ruby"
), JavaScript ("javascript"
, "js"
), CoffeeScript ("coffee"
), Rust ("rc"
, "rs"
, "rust"
), Appollo ("apollo"
, "agc"
, "aea"
), Basic ("basic"
, "cbm"
), Clojure ("clj"
), Css ("css"
), Dart ("dart"
), Erlang ("erlang"
, "erl"
), Go ("go"
), Haskell ("hs"
), Lisp ("cl"
, "el"
, "lisp"
, "lsp"
, "scm"
, "ss"
, "rkt"
), Llvm ("llvm"
, "ll"
), Lua ("lua"
), Matlab ("matlab"
), ML (OCaml, SML, F#, etc) ("fs"
, "ml"
), Mumps ("mumps"
), N ("n"
, "nemerle"
), Pascal ("pascal"
), R ("r"
, "s"
, "R"
, "S"
, "Splus"
), Rd ("Rd"
, "rd"
), Scala ("scala"
), SQL ("sql"
), Tex ("latex"
, "tex"
), VB ("vb"
, "vbs"
), VHDL ("vhdl"
, "vhd"
), Tcl ("tcl"
), Wiki ("wiki.meta"
), XQuery ("xq"
, "xquery"
), YAML ("yaml"
, "yml"
), Markdown ("md"
, "markdown"
), formats ("json"
, "xml"
, "proto"
), "regex"
Didn't found yours? Please, open issue to show your interest & I'll try to add this language in next releases.
List of available themes
- Default (simple light theme)
- Solarized Light
- Monokai
List of available fonts
- Consolas
- CourierNew
- DejaVuSansMono
- DroidSansMonoSlashed
- Inconsolata
- Monaco
Used by
List of apps on Play Store where this library used. Ping me if you want to be here too!
Icon | Application |
---|---|
GeekBrains | |
Codify - Codes On The Go | |
C Programming - 200+ Offline Tutorial and Examples | |
Awesome Android - UI Libraries | |
GitJourney for GitHub | |
Source Code - Lập Trình |
Contribute
- You can add your theme (see ColorTheme class). Try to add some classic color themes or create your own if it looks cool. You can find many of them in different open-source text editors.
- If you are strong in regex, add missed language as shown here. You can find existing regex for some language in different sources of libraries, which plays the same role.
- Various adapters also welcome.
Author
Kirill Biakov
License MIT
Copyright (c) 2016 Kirill Biakov
Permission is hereby granted, free of charge, to any person obtaining a copy
of this software and associated documentation files (the "Software"), to deal
in the Software without restriction, including without limitation the rights
to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
copies of the Software, and to permit persons to whom the Software is
furnished to do so, subject to the following conditions:
The above copyright notice and this permission notice shall be included in all
copies or substantial portions of the Software.
THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
SOFTWARE.
*Note that all licence references and agreements mentioned in the CodeView README section above
are relevant to that project's source code only.