Description
Kotlin's scope functions (let, also, takeIf and takeUnless) and the safe call operator are now available to Java.
K2J-Compat alternatives and similar packages
Based on the "Kotlin" category.
Alternatively, view K2J-Compat alternatives based on common mentions on social networks and blogs.
-
Balloon
:balloon: Modernized and sophisticated tooltips, fully customizable with an arrow and animations for Android. -
kotlin-android-template
Android + Kotlin + Github Actions + ktlint + Detekt + Gradle Kotlin DSL + buildSrc = โค๏ธ -
NotyKT ๐๏ธ
๐ NotyKT is a complete ๐Kotlin-stack (Backend + Android) ๐ฑ application built to demonstrate the use of Modern development tools with best practices implementation๐ฆธ. -
Material Chip View
Material Chip view. Can be used as tags for categories, contacts or creating text clouds -
DrawableToolbox
๐ ๏ธ The missing drawable toolbox for Android. Create drawables programmatically and get rid of the boring and always repeated drawable.xml files. -
Navigation Toolbar for Android
:octocat: Navigation toolbar is a slide-modeled UI navigation controller made by @Ramotion -
Capturable
๐Jetpack Compose utility library for capturing Composable content and transforming it into Bitmap Image๐ผ๏ธ -
Pdf Viewer For Android
A Lightweight PDF Viewer Android library which only occupies around 80kb while most of the Pdf viewer occupies up to 16MB space. -
Carousel Recyclerview
Carousel Recyclerview let's you create carousel layout with the power of recyclerview by creating custom layout manager. -
Pluto Debug Framework
Android Pluto is a on-device debugging framework for Android applications, which helps intercept Network calls, capture Crashes & ANRs, manipulate application data on-the-go, and much more. -
CrunchyCalendar โ awesome calendar widget for android apps
A beautiful material calendar with endless scroll, range selection and a lot more! -
SSComposeCookBook
A Collection of major Jetpack compose UI components which are commonly used.๐๐๐ -
Permission Flow for Android
Know about real-time state of a Android app Permissions with Kotlin Flow APIs. -
SSCustomBottomNavigation
Animated TabBar with native control and Jetpack Navigation support..โจ๐๐ -
Only
:bouquet: An easy way to persist and run code block only as many times as necessary on Android. -
Nextflix-Composable
Includes jetpack compose, navigation, paging, hilt, retrofit, coil, coroutines, flow.. -
EasyPermissions-ktx
๐ Kotlin version of the popular google/easypermissions wrapper library to simplify basic system permissions logic on Android M or higher. -
Compose Compiler Reports to HTML Generator
A utility (Gradle Plugin + CLI) to convert Jetpack Compose compiler metrics and reports to beautified HTML page. -
Events Calendar
Events Calendar is a user-friendly library that helps you achieve a cool Calendar UI with events mapping. You can customise every pixel of the calendar as per your wish and still achieve in implementing all the functionalities of the native android calendar in addition with adding dots to the calendar which represents the presence of an event on the respective dates. It can be done easily, you are just a few steps away from implementing your own badass looking Calendar for your very own project! -
MidJourney Images Compose Multiplatform Mobile Application
This application is developed to display the images created by MidJourney. The application is developed with Compose Multiplatform and works on many platforms including Android and iOS platforms. -
SSCustomEditTextOutLineBorder
Same as the Outlined text fields presented on the Material Design page but with some dynamic changes. ๐ ๐ -
TimelineView
A customizable and easy-to-use Timeline View library for Android. Works as a RecyclerView decorator (ItemDecoration) -
Vanilla Place Picker
Simple(vanilla) yet 'Do it all' place picker for your place picking needs in Android
WorkOS - The modern identity platform for B2B SaaS
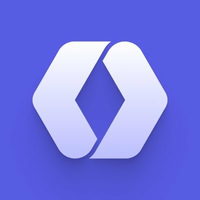
Do you think we are missing an alternative of K2J-Compat or a related project?
README
K2J-Compat
Bringing some of the Kotlin goodness to java
Installation with Android Gradle
// Add K2J-Compat dependency
dependencies {
implementation 'com.github.alexdochioiu:k2j-compat:1.0.1'
}
Usage
1. Kotlin's .let{ }
as .let()
import static com.github.alexdochioiu.k2jcompat.K2JCompat.take;
final String number = take("+44 77 1234 1234")
.let((it) -> "0" + it.substring(4)) // 077 1234 1234
.let((it) -> it.replaceAll(" ", "")) // 07712341234
.unwrap(); // unwraps the result to String
2. Kotlin's ?.let{ }
as ._let()
import static com.github.alexdochioiu.k2jcompat.K2JCompat.take;
final String nullStr = null;
final String number = take(nullStr)
._let((it) -> it.replaceAll(" ", "")) // this code will not run as 'it' is null
.unwrap(); // returns null
3. Kotlin's .also{ }
as .also()
import static com.github.alexdochioiu.k2jcompat.K2JCompat.take;
StringBuilder builder = new StringBuilder();
take(builder).also((it) -> it.append("Hello World!"));
4. Kotlin's ?.also{ }
as ._also()
import static com.github.alexdochioiu.k2jcompat.K2JCompat.take;
StringBuilder builder = null;
take(builder)._also((it) -> it.append("Hello World!"));
// Note: using 'also' instead of '_also' will cause a null pointer exception when trying to append
5. Kotlin's .takeIf{ }
as .takeIf()
import static com.github.alexdochioiu.k2jcompat.K2JCompat.take;
String phoneNumber = "889944";
Integer number = take(phoneNumber)
.takeIf((it) -> it.matches("[0-9]+"))
._let(Integer::parseInt)
.unwrap();
6. Kotlin's ?.takeIf{ }
as ._takeIf()
import static com.github.alexdochioiu.k2jcompat.K2JCompat.take;
String phoneNumber = null;
Integer number = take(phoneNumber)
._takeIf((it) -> it.matches("[0-9]+"))
._let(Integer::parseInt)
.unwrap();
Note: If you use int instead of Integer NPE will be thrown as java will try to unbox a null Integer to int
7. Kotlin's .takeUnless{ }
as .takeUnless()
Similar to takeIf but with inverted logic
8. Kotlin's ?.takeUnless{ }
as ._takeUnless()
Similar to _takeIf but with inverted logic
Known Limitations
- Unlike Kotlin, Java has primitive data types which get boxed/unboxed automatically into objects when needed. However, NPE is thrown when Java tries to unbox a null Object to a primitive data type. This cannot be solved so a custom lint will be created to add a warning when using primitives are used.
*Note that all licence references and agreements mentioned in the K2J-Compat README section above
are relevant to that project's source code only.