Description
Android view library that represents tree structure data.
DynamicTree alternatives and similar packages
Based on the "Kotlin" category.
Alternatively, view DynamicTree alternatives based on common mentions on social networks and blogs.
-
Balloon
:balloon: Modernized and sophisticated tooltips, fully customizable with an arrow and animations for Android. -
kotlin-android-template
Android + Kotlin + Github Actions + ktlint + Detekt + Gradle Kotlin DSL + buildSrc = โค๏ธ -
NotyKT ๐๏ธ
๐ NotyKT is a complete ๐Kotlin-stack (Backend + Android) ๐ฑ application built to demonstrate the use of Modern development tools with best practices implementation๐ฆธ. -
Material Chip View
Material Chip view. Can be used as tags for categories, contacts or creating text clouds -
DrawableToolbox
๐ ๏ธ The missing drawable toolbox for Android. Create drawables programmatically and get rid of the boring and always repeated drawable.xml files. -
Navigation Toolbar for Android
:octocat: Navigation toolbar is a slide-modeled UI navigation controller made by @Ramotion -
Capturable
๐Jetpack Compose utility library for capturing Composable content and transforming it into Bitmap Image๐ผ๏ธ -
Pdf Viewer For Android
A Lightweight PDF Viewer Android library which only occupies around 80kb while most of the Pdf viewer occupies up to 16MB space. -
Carousel Recyclerview
Carousel Recyclerview let's you create carousel layout with the power of recyclerview by creating custom layout manager. -
CrunchyCalendar โ awesome calendar widget for android apps
A beautiful material calendar with endless scroll, range selection and a lot more! -
Pluto Debug Framework
Android Pluto is a on-device debugging framework for Android applications, which helps intercept Network calls, capture Crashes & ANRs, manipulate application data on-the-go, and much more. -
SSComposeCookBook
A Collection of major Jetpack compose UI components which are commonly used.๐๐๐ -
Permission Flow for Android
Know about real-time state of a Android app Permissions with Kotlin Flow APIs. -
SSCustomBottomNavigation
Animated TabBar with native control and Jetpack Navigation support..โจ๐๐ -
Only
:bouquet: An easy way to persist and run code block only as many times as necessary on Android. -
Nextflix-Composable
Includes jetpack compose, navigation, paging, hilt, retrofit, coil, coroutines, flow.. -
EasyPermissions-ktx
๐ Kotlin version of the popular google/easypermissions wrapper library to simplify basic system permissions logic on Android M or higher. -
Compose Compiler Reports to HTML Generator
A utility (Gradle Plugin + CLI) to convert Jetpack Compose compiler metrics and reports to beautified HTML page. -
Events Calendar
Events Calendar is a user-friendly library that helps you achieve a cool Calendar UI with events mapping. You can customise every pixel of the calendar as per your wish and still achieve in implementing all the functionalities of the native android calendar in addition with adding dots to the calendar which represents the presence of an event on the respective dates. It can be done easily, you are just a few steps away from implementing your own badass looking Calendar for your very own project! -
MidJourney Images Compose Multiplatform Mobile Application
This application is developed to display the images created by MidJourney. The application is developed with Compose Multiplatform and works on many platforms including Android and iOS platforms. -
SSCustomEditTextOutLineBorder
Same as the Outlined text fields presented on the Material Design page but with some dynamic changes. ๐ ๐ -
TimelineView
A customizable and easy-to-use Timeline View library for Android. Works as a RecyclerView decorator (ItemDecoration) -
Vanilla Place Picker
Simple(vanilla) yet 'Do it all' place picker for your place picking needs in Android
InfluxDB - Power Real-Time Data Analytics at Scale
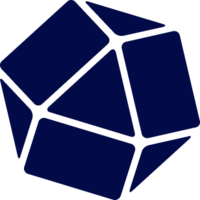
Do you think we are missing an alternative of DynamicTree or a related project?
README
Android view library that represents tree structure data.
Sample
Install
Gradle
- Add the JitPack repository to your project level build.gradle file
allprojects {
repositories {
...
maven { url 'https://jitpack.io' }
}
}
- Add the dependency to your app level build.gradle file
dependencies {
implementation "com.github.wooooooak.DynamicTree:$version"
implementation "androidx.recyclerview:recyclerview:$recyclerViewVersion"
}
The latest version is 1.0.0. Checkout here
Usage
1. Set resource files (layout and color)
layout.xml
<androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
...
>
<wooooooak.com.library.view.DynamicTreeView
android:id="@+id/dt_view"
android:layout_width="match_parent"
android:layout_height="match_parent"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent"
app:dt_animation_duration="250"
app:dt_depth_limit="3"
app:dt_list_page_margin="8dp"
app:dt_stack_view_color_array="@array/dtColorArray" />
</androidx.constraintlayout.widget.ConstraintLayout>
dt_list_page_margin : determines how overlapping the view looks. If the page goes beyond the
dt_depth_limit
, the new data is rendered but the depth shown to the user is still the depth you set.dt_stack_view_color_array : "dt_stack_view_color_array" should be one more than "dt_depth_limit".
colors.xml
<?xml version="1.0" encoding="utf-8"?>
<resources>
...
<array name="dtColorArray">
<item>#74c0fc</item>
<item>#a5d8ff</item>
<item>#d0ebff</item>
<item>#e7f5ff</item>
</array>
</resources>
2. Prepare Tree Data
We need a list of tree. And Each node must implement UniqueKey for search algorithm.
// For Eaxample
data class Node(
override val uniqueKey: String, // you should override
override val child: List<Node>?, // you should override
val personInfo: Person? // or anything more
) : UniqueKey
data class Person(
val age: Int,
val number: String
)
Finally, DynamicTree uses the node list.
dt_view.treeData = listOf<Node>(...)
3. Make OrgGroupAdapter
class TestAdapter: DynamicTreeAdapter<Node>(
R.layout.item_group, // layout id for Group item view
R.layout.item_last_node // layout id for Last node item view
) {
override fun isTargetNode(item: Node): Boolean {
TODO("Used to check whether it is the last node or not")
}
override fun bindDefultLastNodeView(view: View, item: Node) {
TODO("Bind default last node view ")
}
override fun bindDefaultGroupView(view: View, item: Node) {
TODO("Bind default group item view ")
}
override fun bindClickedGroupView(view: View, item: Node) {
TODO("Bind group item view when clicked")
}
override fun onClickGroupItem(groupItemView: View, item: Node) {
TODO("Called when group item clicked")
}
override fun onClickLastNodeItem(lastNodeItemView: View, item: Node) {
TODO("Called when last node clicked")
}
}
Note that DynamicTreeAdapter takes the UniquKey type as generic.
4. In your Activity or Fragment
override fun onCreate(savedInstanceState: Bundle?) {
...
val treeList: List<Node> = ...
dt_view.treeData = treeList
dt_view.orgAdapterGenerator = { MyOrgGroupAdapter() }
dt_view.render()
}
override fun onBackPressed() {
if (dt_view.canGoBack()) {
dt_view.goBack()
} else {
super.onBackPressed()
}
}
You can clone this project and run the demo with sample data immediately.
License
Copyright 2020 wooooooak (Yongjun LEE)
Licensed under the Apache License, Version 2.0 (the "License");
you may not use this file except in compliance with the License.
You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software
distributed under the License is distributed on an "AS IS" BASIS,
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
See the License for the specific language governing permissions and
limitations under the License.
*Note that all licence references and agreements mentioned in the DynamicTree README section above
are relevant to that project's source code only.